Tip Object
Description
The Tip object describes a single tip of a probe. All of its properties are read-only.
Object Model
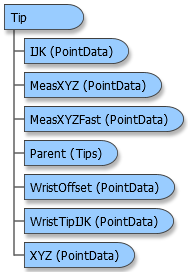
Example
using System; using System.Diagnostics; namespace ProcessTipOffsets { public static class Utilities { public static bool IsProcessOpen(string name) { foreach (Process clsProcess in Process.GetProcesses()) { if (clsProcess.ProcessName.Equals(name, StringComparison.InvariantCulture)) { return true; } } return false; } } class Program { public static PCDLRN.Application GetPCDApp() { System.Type objPCDType = System.Type.GetTypeFromProgID("PCDLRN.Application"); return (PCDLRN.Application)System.Activator.CreateInstance(objPCDType); } private static void ErrorMessage(string s) { Console.WriteLine(s); } static void Main(string[] args) { string probeName = "PROBE_HPL"; if (args.Length > 0 && args[1] == "--help") { Console.WriteLine("PcdProcessTips [<ProbeName>|--help]"); return; } if (args.Length > 0) { probeName = args[0]; } if (!Utilities.IsProcessOpen("PCDLRN")) { ErrorMessage("PCDMIS application cannot be found"); return; } PCDLRN.Application app = GetPCDApp(); if (app == null) { ErrorMessage("PCDMIS application cannot be instantiated (found)"); return; } PCDLRN.PartProgram pp = app.ActivePartProgram; if (pp == null) { ErrorMessage("PCDMIS Active Part Program not found"); return; } var PcProbes = pp.Probes; var PcProbe = PcProbes.Item(probeName); if (PcProbe == null) { ErrorMessage("Probe not found: " + probeName); return; } var PcTips = PcProbe.Tips; Console.WriteLine("Probe name: {0}", probeName); int count = PcTips.Count; for (var it = 0; it < count; it += 1) { var PcTip = PcTips.Item(it+1); string name = PcTip.ID; int pc = PcTip.GetGeneralParametersCount(); Console.WriteLine("TipName: {0}", name); if (pc == 12) { for (var ip = 0; ip < pc; ip += 3) { double v = PcTip.GetGeneralParameters(ip); double v1 = PcTip.GetGeneralParameters(ip + 1); double v2 = PcTip.GetGeneralParameters(ip + 2); Console.WriteLine("{0} {1} {2}", v, v1, v2); } } else { Console.WriteLine("Error: wrong number of general parameters for the tip (must be 12)", pc); } Console.WriteLine(); } PcProbes = null; } } }
See Also